Software design is the first stage of the Software Design Lifecycle. It defines:
- How the individual modules and components of a system will be designed
- Detailed software properties
- Specifications that will help developers implement the software
- How all components, modules, functions, and so on are being built
Software architecture is the blueprint for a software system and the highest level of a software design. It defines:
- Which elements and components need to be in the system
- Which components need to interact
- The type of environment the software needs to operate
Software development is a complex process. Two important parts of the process include software design and software architecture. Sometimes it can be hard to tell the difference between the two.
Basically, software design is about the individual modules and components of the software, and software architecture is about the overall structure that these components fit into.
In this article, we’ll delve more deeply into software design vs. software architecture. We’ll answer what is software design, what is software architecture, and review what makes them similar and what sets them apart.
What is software architecture?
Like a blueprint of a building, a bridge, or any other kind of structure, software architecture is used to organize and conceptualize a system. It includes a definition of which elements and components need to be in the system, which components need to interact with each other, and what type of environment the software needs to operate.
Software architecture defines the structure and constraints that the software developers will need to work in. It includes the documentation, charts, diagrams, and anything used to facilitate communications with stakeholders.
Characteristics of software architecture
Architecture characteristics define the software’s requirements and what it is expected to do. Some of the characteristics shared by software architectures include:
A description of the overall system setup: This includes the structure of the software you want to build. To make it easier for stakeholders to understand, you might want to create a visual representation with diagrams and charts. Visuals are a great way to show relationships between components and subsystems. They give everybody involved insight into the architecture and give you perspective as you analyze the structure and look for ways to improve your structure or plan an expansion to an existing system.
A definition of fundamental elements: Software architecture defines the core set of elements and properties that are required to build the system. It does not document every element in detail. It simply identifies the structures that are required to build the software’s core functionality. For example, a web browser and a web server describe the core elements needed for a user to interact with the internet.
A description of high-level structures: The development teams need to make decisions about the high-level structure that describe things like the system availability, performance, ability to scale, system reliability and fault tolerance, configuration and support, and monitoring and maintenance.
A description of what is being built: You are likely building software or a system to address the needs and requirements of stakeholders. But you can’t always fully develop everything that the stakeholders ask for. A description of what you are building can help you manage stakeholder expectations. Use diagrams, flowcharts, and process documents to keep stakeholders informed and to avoid feature and scope creep.
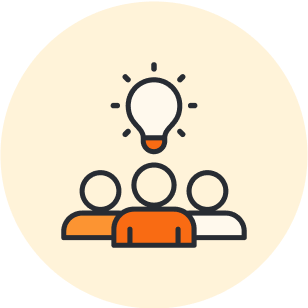
Learn how to draw your own architectural diagrams.
Read moreSoftware architecture patterns
Developers often run into similar problems while working on a project. Software architecture patterns give developers a way to solve these problems whenever they come up. This allows them to get the same structural output on any given project.
Software architecture patterns are important because they help developers to be more productive and efficient. An understanding of the patterns means that software developers can easily move from project to project with very little onboarding.
While there are several different software architecture patterns, we are going to look at the following three:
- Serverless architecture
- Event-driven architecture
- Microservices architecture
Serverless architecture pattern
This pattern can be used to build software and services without managing the infrastructure. A third-party is used to manage servers, the backend, and other services. This lets you focus on fast, continuous software delivery. When you don’t have to worry about managing the infrastructure and planning for expansion, you have more time to look at the value that can be added to your software and services.
Event-driven software architecture
This type of architecture relies on events to trigger actions, communications, and other services in a decoupled system. An event can be anything that changes the current state. Think about when a customer adds bank information to the payment options section in their account on an e-commerce website. The event can carry the state, like when a purchase is completed. Or the event can be an identifier, like when a notification is sent that an order has been placed successfully.
Event-driven architecture includes event producers and event consumers. The producers detect events and transmit them to the consumer events. The consumer event might process the event, or it might only be impacted by the event.
Microservices software architecture
Microservices are multiple applications that work interdependently. These microservices are developed independently and each is designed to solve specific problems or to perform specific tasks. But their functionality is also designed to communicate with each other and to be entwined so they can work together to achieve business goals.
Because each microservice is developed separate from the others, development is streamlined and deployment is easier. This also increases your ability to quickly scale to meet customer expectations.
What is software design?
While the software architecture identifies the components and elements that need to be included in the software, the software design focuses on how the software will be built.
Software design is one of the initial phases of the software development life cycle. In this phase you analyze and identify the methods that your developers will use. Additionally, you define how the software will be built according to stakeholder and customer requirements.
The software design defines:
- How the individual modules and components will be designed
- Detailed software properties
- Specifications that will help developers implement the software
- How all components, modules, functions, and so on are being built
Characteristics of software design
To produce high-quality software, you need a high-quality design. High-quality designs share the following common characteristics:
-
Correctness: Your design needs to be correct so you can implement everything that is identified as your software requirements.
-
Understandability: A design that is easy to understand is easier for developers to work with. The design should be clear and as self-explanatory as possible. If you have to spend too much time verbally explaining what needs to be done, then your design is a hindrance to the development of the software.
-
Efficiency: If the design does not describe efficient or useful software, then the software you produce won’t have the value that your customers expect.
-
Maintainability: Your design will probably go through changes. So it needs to be easy to maintain and stored in an easily accessible location so team members can quickly see any changes made.
Software design patterns
A software design pattern describes the problem, the solution, when you might want to apply the solution, and includes hints and examples for implementation.
There are multiple software design patterns that are categorized into one of the following three design pattern types:
-
Creational: These patterns deal with the way objects are created. They create objects in a controlled manner to reduce complexity and instability. Software design patterns in this category include:
-
Factory pattern: Defines an interface or an abstract class for creating a single object.
-
Adapter pattern: Used to create a bridge between incompatible objects so they will work in environments that they weren’t originally intended to work in.
-
Singleton pattern: Ideal when you need only one instance of an object to exist in the application.
-
-
Structural: These patterns are concerned with class composition and structure. They identify relationships to simplify the structure. Structural design patterns include:
-
Decorator pattern: Gives you a way to add new behavior to an object by placing the behavior inside a wrapper.
-
Facade pattern: Uses a simple interface that hides complex details such as legacy code and third party libraries.
-
Proxy pattern: Uses a class as an interface into something else.
-
-
Behavioral: These patterns identify how objects communicate with other objects. They look for common patterns that will make communication more flexible and ease interaction between objects.
-
Blackboard pattern: Coordinates different systems that need to work together or that need to work in a sequence.
-
Command pattern: Lets you configure a class with a command object that can perform a specific request.
-
The SOLID principle
The SOLID principle is an acronym for five design principles that can help you to create more flexible, accessible software designs that are simple to maintain. These principles are:
-
Single responsibility principle: Each class in a software program should have a single responsibility.
-
Open closed principle: A class should be open for extension, meaning that you should be able to add new functionality to it. But the class should also be closed to modification, meaning that the changes you make should not break existing code.
-
Liskov substitution principle: This principle states that if object S is a subtype of object T, then objects of type T may be replaced with objects of subtype S.
-
Interface segregation principle: This principle states that you should not use new methods to attach additional functionality to an existing interface. Instead, create a new object that can implement multiple interfaces.
-
Dependency inversion principle: This principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
How are software architecture and design related?
While software architecture and design should be considered as two separate stages in the software development process, they do tend to overlap. For example, they’re both concerned with the relationships and interactions of various components and elements in the software structure. But they differ in their approach. Software architecture is primarily concerned with what the components and relationships are, and software design focuses on how the components interact.
Software architecture and design are two separate parts of one process that depend on each other for success.
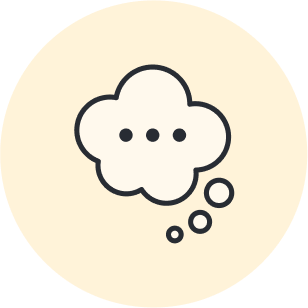
If you're ready to take on the software design piece of the process, get started with a software design document.
Learn how to create oneAbout Lucidchart
Lucidchart, a cloud-based intelligent diagramming application, is a core component of Lucid Software's Visual Collaboration Suite. This intuitive, cloud-based solution empowers teams to collaborate in real-time to build flowcharts, mockups, UML diagrams, customer journey maps, and more. Lucidchart propels teams forward to build the future faster. Lucid is proud to serve top businesses around the world, including customers such as Google, GE, and NBC Universal, and 99% of the Fortune 500. Lucid partners with industry leaders, including Google, Atlassian, and Microsoft. Since its founding, Lucid has received numerous awards for its products, business, and workplace culture. For more information, visit lucidchart.com.
Related articles
How to design software architecture: Top tips and best practices
Check out these helpful software architecture design tips and best practices for building your own software architecture.
How to create software design documents
A software design document can help keep you and your team on track from the start of a project to the last lines of code. Learn everything from the basics to best practices of creating software design documents.
How to eliminate pain points of software design and architecture
The process of software development can go smoothly or be fraught with problems. Use these best practices to reduce the risk of your project running over budget and out of scope.
How to break your software design down into actionable next steps
Starting with your requirements and software architecture design, your team can plan consistent and accurate steps for your engineers to follow all the way to a successful project. Here's how.